本文概述
借助webkit, 你可以根据需要使用CSS自定义应用程序的UI。可以在你的应用程序窗口内创建花式通知, 但是有时你可能想要向用户通知某些内容, 即使该内容不是一开始就使用该应用程序或已被最小化。
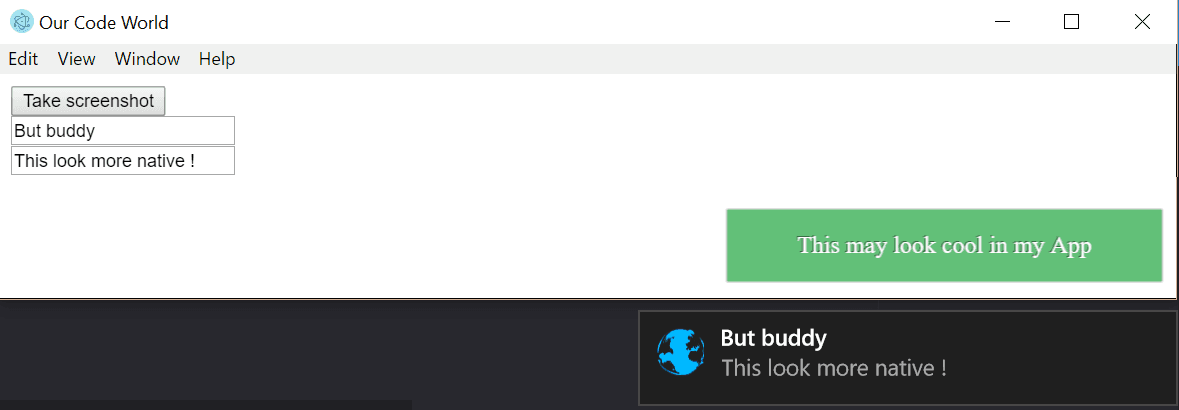
这就是为什么我们需要访问操作系统的通知系统, 该通知系统允许你在任何情况下都可以向屏幕上的用户发送消息, 尽管他们正在使用其他应用程序。
但是请记住, 多余的东西都是不好的。你可能需要注意向用户显示此消息的频率, 并请求这样做的许可, 因为过度使用本机通知会令人烦恼和恼怒。
可能你会问自己:为什么不使用webkitNotification API?你也可以将此API与Electron一起使用, 但是不适用于Windows。它可以在Linux和MacOS上使用, 但是它的外观和外观并不相同, 因此建议你在Electron应用程序中使用node-notifier插件。
节点通知程序是用于发送跨平台系统通知的Node.js模块。使用Mac的Notification Center, 使用Linux的notify-osd / libnotify-bin, Windows 8/10的Toasters或Windows早期版本的任务栏Balloons。如果这些条件都不满足, 则使用Growl。
实现
为了实现我们的目标, 我们将使用可以通过以下命令在你的项目中下载的节点通知程序库:
npm install --save node-notifier
在此处访问项目的官方资源库。成功安装后, 你将使用require(‘node-notifier’)访问通知程序。
重要说明:Electron本身(最新版本)为你提供Notification API, 该API在操作系统中发送简单的通知(与节点通知程序相同的方式)。你可以使用以下代码段尝试使用它。
这三个操作系统都为应用程序提供了向用户发送通知的方法。 Electron方便地允许开发人员使用HTML5 Notification API发送通知, 并使用当前运行的操作系统的本机通知API进行显示。
注意:由于这是HTML5 API, 因此仅在渲染器过程中可用。
// You can use console.log(notification); to see more available properties
var notification = new Notification('Title', {
body: 'Lorem Ipsum Dolor Sit Amet', title:"Hello", icon:'C:/images/icon.png', // To prevent sound
//silent:true, });
notification.addEventListener("click", function(){
alert("Clicked");
}, false);
notification.addEventListener("show", function(){
alert("Shown :3");
}, false);
notification.addEventListener("error", function(e){
alert("Error :c");
}, false);
notification.addEventListener("close", function(){
alert("Closed");
}, false);
如果你认为自己想要更多, 请继续阅读节点通知程序的用法。
通知所有
现在, 你只需要开始使用通知程序, 以下代码段显示了一条带有声音和图标的简单消息。
注意:notify方法是跨平台的, 如果你阅读文档, 则可以对每个平台进行更深入的自定义。
const notifier = require("node-notifier");
var onError = function(err, response){
console.error(err, response);
};
notifier.notify({
message: "This is the body of the notification.", title: "This will be the title of the notification", // Special sound
// Case Sensitive string for location of sound file, or use one of OS X's native sounds
// Only Notification Center or Windows Toasters
sound: true, //"Bottle", // The absolute path to the icon of the message
// (doesn't work on balloons)
// If not found, a system icon will be shown
icon : "C:/images/ocw-logo.png", // Wait with callback (onClick event of the toast), until user action is taken against notification
wait:true
}, onError);
notifier.on('click', function (notifierObject, options) {
// Triggers if `wait: true` and user clicks notification
alert("Callback triggered");
});
notify方法是跨平台标准用法, 具有跨平台回退。此方法将在所有平台上以某种方式工作。
但是, notifier允许你根据需要根据每个平台进行自定义, 请参见存储库中有关自定义的详细报告。
视窗
烤面包机模块仅适用于Windows> = 8。
本机Windows 8通知中的图像有一些限制:该图像必须是PNG图像, 并且不能超过1024×1024 px或超过200Kb。你还需要使用绝对路径来指定图像。这些限制归因于Toast通知系统。一个很好的技巧是使用类似path.join或path.delimiter之类的东西来进行跨平台的路径操作。
Windows 10注意:你可能必须激活横幅通知才能显示烤面包。
const WindowsToaster = require('node-notifier').WindowsToaster;
var notifier = new WindowsToaster({
withFallback: false, // Fallback to Growl or Balloons?
customPath: void 0 // Relative path if you want to use your fork of toast.exe
});
notifier.notify({
title: "Title", message: "Message", icon: "c:/path/image.png", // Absolute path to Icon
sound: true, // true | false.
wait: false, // Wait for User Action against Notification
}, function(error, response) {
console.log(response);
});
对于早期的Windows版本, 将使用任务栏气球(除非激活了后备功能并且正在运行Growl)。
苹果系统
Native Notification Center需要Mac OS X 10.8或更高版本。如果你使用的是较早的版本, 那么Growl将是你的后备。如果未安装Growl, 则回调中将返回错误。
const NotificationCenter = require('node-notifier').NotificationCenter;
var notifier = new NotificationCenter({
withFallback: false, // Use Growl Fallback if <= 10.8
customPath: void 0 // Relative path if you want to use your fork of terminal-notifier
});
notifier.notify({
'title': "Hello world", 'subtitle': void 0, 'message': void 0, 'sound': false, // Case Sensitive string for location of sound file, or use one of OS X's native sounds (see below)
'icon': 'Terminal Icon', // Absolute Path to Triggering Icon
'contentImage': void 0, // Absolute Path to Attached Image (Content Image)
'open': void 0, // URL to open on Click
'wait': false // Wait for User Action against Notification
}, function(error, response) {
console.log(response);
});
声音可以是以下之一:巴索, 打击, 瓶子, 青蛙, 放克, 玻璃, 英雄, 莫尔斯, 砰, 砰, 流行, 咕r声, 纯水, 潜艇, 叮叮当。如果声音完全正确, 则使用Bottle。
请参阅特定的通知中心示例。
Electron的已知问题
在Electron打包中如果将Electron应用程序打包为asar, 则会发现node-notifier将无法加载。由于asar的工作方式, 你无法从asar内部执行二进制文件。作为一个简单的解决方案, 将应用程序打包为asar时, 请确保–unpack node-notifier的供应商文件夹, 以便该模块仍然可以访问通知二进制文件。为此, 你可以使用以下命令来这样做:
asar pack . app.asar --unpack "./node_modules/node-notifier/vendor/**"
玩得开心
评论前必须登录!
注册