本文概述
必须先将其解压缩, 然后才能开始实际使用zip文件中的内容(文件)。在本文中, 你将学习如何使用Electron Framework和decompress-zip模块解压缩.zip文件。
要求
为了在Electron中解压缩文件, 你需要decompress-zip模块, 该模块允许你使用几行代码从ZIP存档中提取文件。要在项目中安装decompress-zip文件, 请在命令提示符下执行以下命令:
npm install decompress-zip
安装后, 你将可以使用require(‘decompress-zip’)在代码中要求该模块。请访问Github中的模块存储库以获取更多信息。
实现
使用decompress-zip, 你将能够执行2个任务:解压缩.zip文件并列出.zip文件的内容。
解压缩(解压缩.zip文件)
要解压缩a.zip文件, 我们将使用unzipper.extract方法。此方法提取ZIP存档文件的内容, 并返回带有两个可能事件的EventEmitter:错误时触发错误, 提取完成后提取。
传递给extract事件的值是每个文件及其压缩方式的基本日志。要解压缩文件, 你只需要提供zip文件的当前路径和目标目录:
var ZIP_FILE_PATH = "C:/path/to/file/myzipfile.zip";
var DESTINATION_PATH = "C:/desktop/examplefolder";
var unzipper = new DecompressZip(ZIP_FILE_PATH);
// Add the error event listener
unzipper.on('error', function (err) {
console.log('Caught an error', err);
});
// Notify when everything is extracted
unzipper.on('extract', function (log) {
console.log('Finished extracting', log);
});
// Notify "progress" of the decompressed files
unzipper.on('progress', function (fileIndex, fileCount) {
console.log('Extracted file ' + (fileIndex + 1) + ' of ' + fileCount);
});
// Start extraction of the content
unzipper.extract({
path: DESTINATION_PATH
// You can filter the files that you want to unpack using the filter option
//filter: function (file) {
//console.log(file);
//return file.type !== "SymbolicLink";
//}
});
如果要在解压缩时过滤文件, 如前所示, 可以依靠unzipper.extract方法中的filter属性(如果返回的值为true, 则将解压缩文件)。文件对象(在过滤器回调中)包含以下所有属性:
{
"_offset":40, "_maxSize":34348, "parent":".", "filename":"image4.png", "path":"image4.png", "type":"File", "mode":438, "compressionMethod":8, "modified":"2016-10-18T14:25:12.000Z", "crc32":1376504589, "compressedSize":34348, "uncompressedSize":34381, "comment":"", "flags":{
"encrypted":false, "compressionFlag1":false, "compressionFlag2":false, "useDataDescriptor":false, "enhancedDeflating":false, "compressedPatched":false, "strongEncryption":false, "utf8":false, "encryptedCD":false
}
}
以下示例显示了使用Electron的固有对话框进行decompress-zip的基本实现。
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Electron Zip Uncompress Files</title>
</head>
<body>
<input type="button" id="select-file" value="Select .zip file" />
<script>
var DecompressZip = require('decompress-zip');
var app = require('electron').remote;
var dialog = app.dialog;
(function () {
function uncompress(ZIP_FILE_PATH, DESTINATION_PATH){
var unzipper = new DecompressZip(ZIP_FILE_PATH);
// Add the error event listener
unzipper.on('error', function (err) {
console.log('Caught an error', err);
});
// Notify when everything is extracted
unzipper.on('extract', function (log) {
console.log('Finished extracting', log);
});
// Notify "progress" of the decompressed files
unzipper.on('progress', function (fileIndex, fileCount) {
console.log('Extracted file ' + (fileIndex + 1) + ' of ' + fileCount);
});
// Unzip !
unzipper.extract({
path: DESTINATION_PATH
});
}
document.getElementById("select-file").addEventListener("click", () => {
// Select the .zip file
dialog.showOpenDialog({
title:"Select the .zip file to decompress"
}, function (fileNames) {
// fileNames is an array that contains the selected file
if(fileNames === undefined){
console.log("No file selected");
return;
}else{
// Select destination folder
dialog.showOpenDialog({
title:"Select destination folder where the content should be decompressed", properties: ["openDirectory"]
}, function (folderPaths) {
// folderPaths is an array that contains all the selected paths
if(fileNames === undefined){
console.log("No destination folder selected");
return;
}else{
// Proceed to decompress
uncompress(fileNames[0], folderPaths[0]);
}
});
}
});
}, false);
})();
</script>
</body>
</html>
前面的代码将创建一个带有按钮的简单应用程序, 该按钮允许你选择要解压缩的.zip文件:
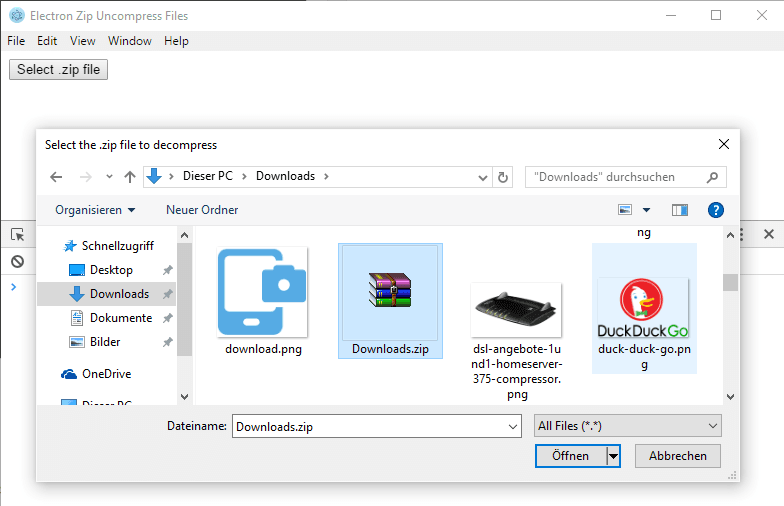
你可以(如果需要)通过在文件浏览器的初始化时添加滤镜属性来仅过滤文件浏览器中的.zip文件:
dialog.showOpenDialog({
title:"Select the .zip file to decompress", filters: [
{name: '.ZIP Files', extensions: ['zip']}, {name: 'All Files', extensions: ['*']}
]
}, (fileNames) => {
// The rest of the code here
});
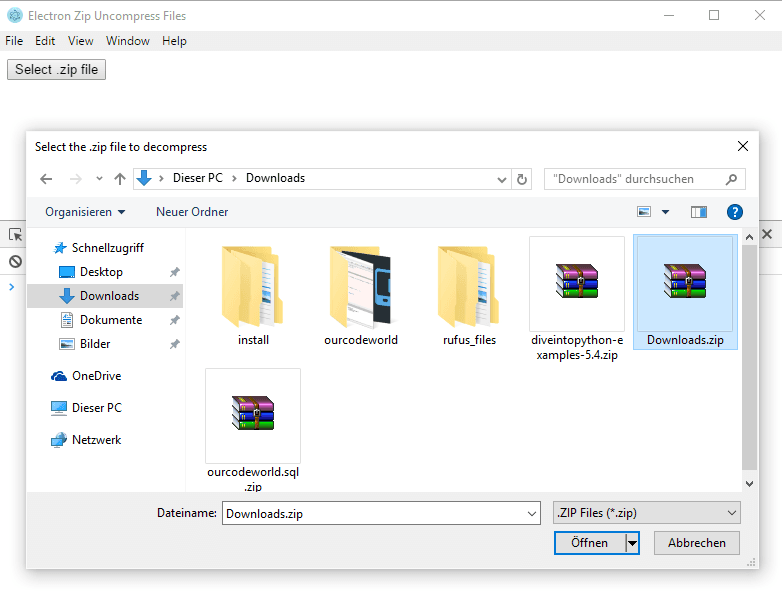
然后, 它将文件的路径存储在一个变量中, 并将再次启动一个对话框, 该对话框允许你选择一个应该解压缩zip文件内容的文件夹:
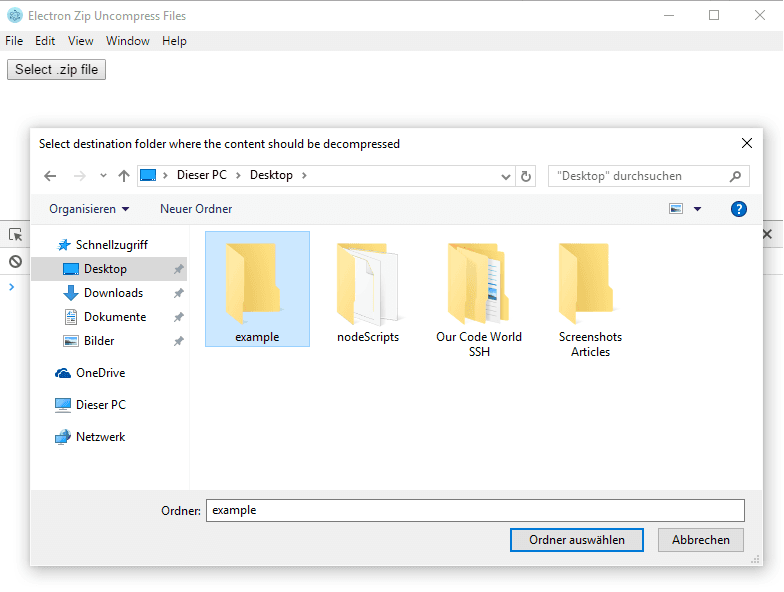
根据之前的操作, Downloads.zip文件将在Desktop / example文件夹中解压缩。选择文件夹后, 解压缩将开始在控制台中显示进度并将文件保存在目标文件夹中:
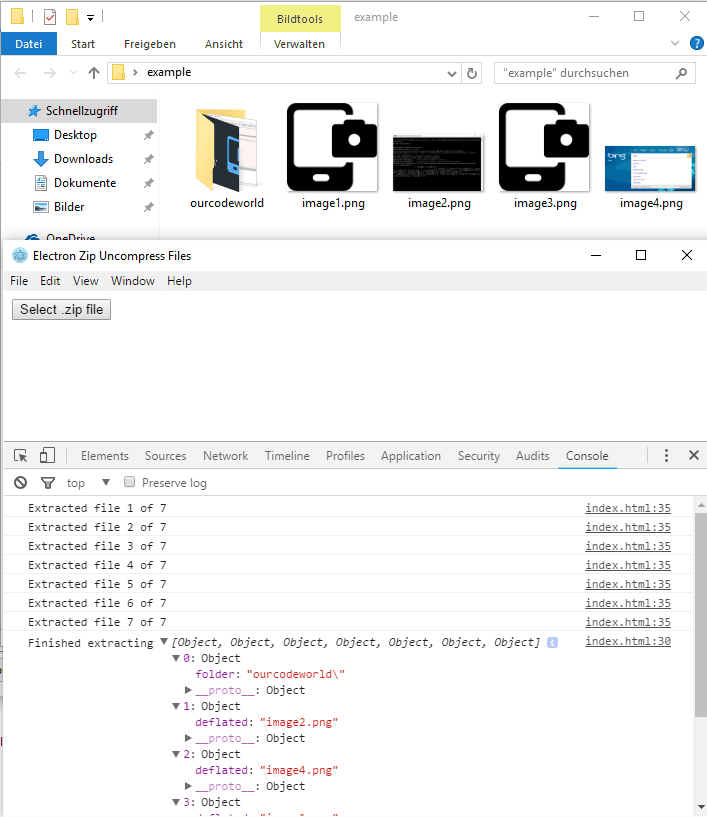
列出.zip文件内容
你可以使用unzipper.list方法列出文件的内容而无需直接解压缩。列表方法与提取类似, 除了成功事件列出事件的数据外。此外, 检索到的数组提供文件的路径, 这意味着实际上没有文件被提取。 list方法不需要参数。
以下代码段应
var ZIP_FILEPATH = "C:/path/to/file/myzipfile.zip";
// Require DecompressZip module
var DecompressZip = require('decompress-zip');
// Declare the unzipper class
var unzipper = new DecompressZip(ZIP_FILEPATH);
// Add the error event listener
unzipper.on('error', (err) => {
console.log('Caught an error', err);
});
// Add the list event listener that will be triggered when the unzipper can list the content
unzipper.on('list', (files) => {
// Show the array of items in the console
console.log(files);
});
// Trigger to list the content of the zip file
unzipper.list();
以下Electron版本的HTML文档显示了如何列出.zip文件的内容(拖放.zip文件以在控制台中列出内容)。
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Electron Zip List Files</title>
<style>
#drag-file {
background-color: blue;
color:white;
text-align: center;
width:300px;
height:300px;
}
</style>
</head>
<body>
<div id="drag-zip">
<p>Drag your zip files here to list content on the console</p>
</div>
<script>
var DecompressZip = require('decompress-zip');
(function () {
var holder = document.getElementById('drag-zip');
holder.ondragover = holder.ondragleave = holder.ondragend = () => {
return false;
};
holder.ondrop = (e) => {
e.preventDefault();
var firstFile = e.dataTransfer.files[0];
if(!firstFile){
console.log("No file given");
return;
}
var unzipper = new DecompressZip(firstFile.path);
unzipper.on('error', (err) => {
console.log('Caught an error', err);
});
unzipper.on('list', (files) => {
console.log('The zip file contains:');
console.log(files);
});
unzipper.list();
return false;
};
})();
</script>
</body>
</html>
以上示例在Electron中的实现应为:
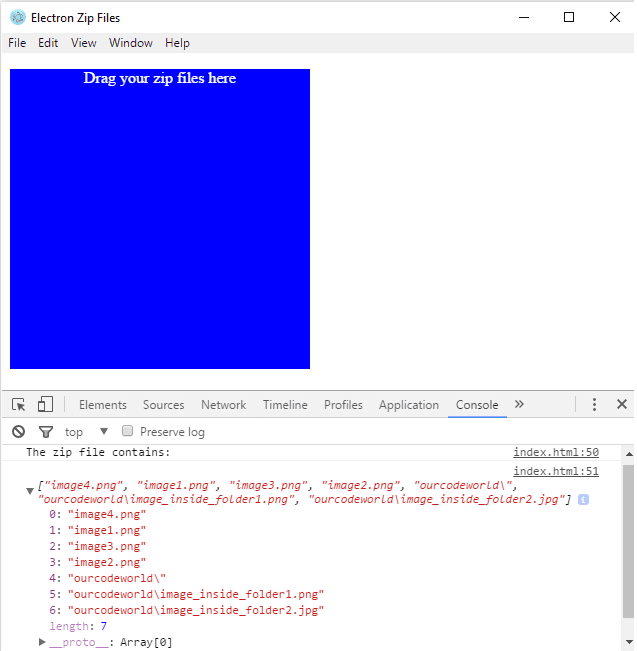
如你所见, 使用Bower创建的此模块, 解压缩.zip文件非常容易。玩得开心 !
评论前必须登录!
注册