本文概述
实现
MainWindow类构造函数将一个对象作为第一个参数。为了使窗口透明, 我们需要分别提供transparent和frame属性, 分别为true和false:
const {BrowserWindow} = require('electron')
let win = new BrowserWindow({
width: 800, height: 600, transparent:true, frame: false
})
win.show()
最好, 如果透明窗口是唯一的且是初始的, 则应在main.js文件中进行更改:
function createWindow () {
// Create the browser window.
mainWindow = new BrowserWindow({
width: 800, height: 600, transparent: true, frame:false
})
// and load the index.html of the app.
mainWindow.loadURL(`file://${__dirname}/index.html`)
// Open the DevTools.
//mainWindow.webContents.openDevTools()
// Emitted when the window is closed.
mainWindow.on('closed', function () {
// Dereference the window object, usually you would store windows
// in an array if your app supports multi windows, this is the time
// when you should delete the corresponding element.
mainWindow = null
})
}
那应该已经使你的窗口透明。将以下标记设置为index.html文件的内容:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Transparent Window</title>
</head>
<body>
<div>
<img src="http://ourcodeworld.com/resources/img/ocw-empty.png" width="300" height="300"/>
</div>
</body>
</html>
并使用npm start执行项目, 你的应用程序将如下所示:

如你所见, 该窗口是完全透明的, 并且你可以在后台看到VSCode。
局限性
- 你不能单击透明区域。我们将引入一个API来设置窗口形状以解决此问题, 有关详细信息, 请参见该问题。
- 透明窗口不可调整大小。将resizable设置为true可能会使透明窗口在某些平台上停止工作。
- 模糊过滤器仅适用于网页, 因此无法将模糊效果应用于窗口下方的内容(即在用户系统上打开的其他应用程序)。
- 在Windows操作系统上, 禁用DWM时透明窗口将不起作用。
- 在Linux上, 用户必须在命令行中添加–enable-transparent-visuals –disable-gpu以禁用GPU并允许ARGB生成透明窗口, 这是由上游错误导致的, 该错误是Alpha通道在某些NVidia上不起作用Linux上的驱动程序。
- 在Mac上, 本机窗口阴影不会显示在透明窗口上。
重要笔记
如果开发人员工具区域可见, 则该窗口将不再透明。如果隐藏或未显示, 则将保留透明效果。
可能没有人想要不能移动的静态窗口, 因此可以使用CSS将拖动功能拖放到元素上:
.draggable-area{
-webkit-app-region: drag;
}
通过将draggable-area类添加到任何元素, 它将允许用户从该点拖动整个窗口。在某些平台上, 可拖动区域将被视为非客户端框架, 因此, 当你右键单击它时, 将弹出一个系统菜单。为了使上下文菜单在所有平台上都能正常运行, 你永远不要在可拖动区域使用自定义上下文菜单。
例子
以下文档将创建一个非常简单的透明窗口, 其中包含几个按钮操作和一个可拖动区域:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Transparent Window</title>
<style>
.draggable-area{
width:300px;
height:300px;
background-color:blue;
color:white;
-webkit-app-region: drag;
}
</style>
</head>
<body>
<div>
<img src="http://ourcodeworld.com/resources/img/ocw-empty.png" width="300" height="300"/>
<div>
<input type="button" id="close" value="Close app"/>
<input type="button" id="devtools" value="Open DEV Tools"/>
<div class="draggable-area">
Drag the window here
</div>
</div>
</div>
<script>
var app = require('electron').remote;
// Close app
document.getElementById("close").addEventListener("click", () => {
app.BrowserWindow.getFocusedWindow().close();
}, false);
// Close app
document.getElementById("devtools").addEventListener("click", () => {
app.BrowserWindow.getFocusedWindow().openDevTools();
}, false);
</script>
</body>
</html>
先前的标记应生成一个类似以下的应用:
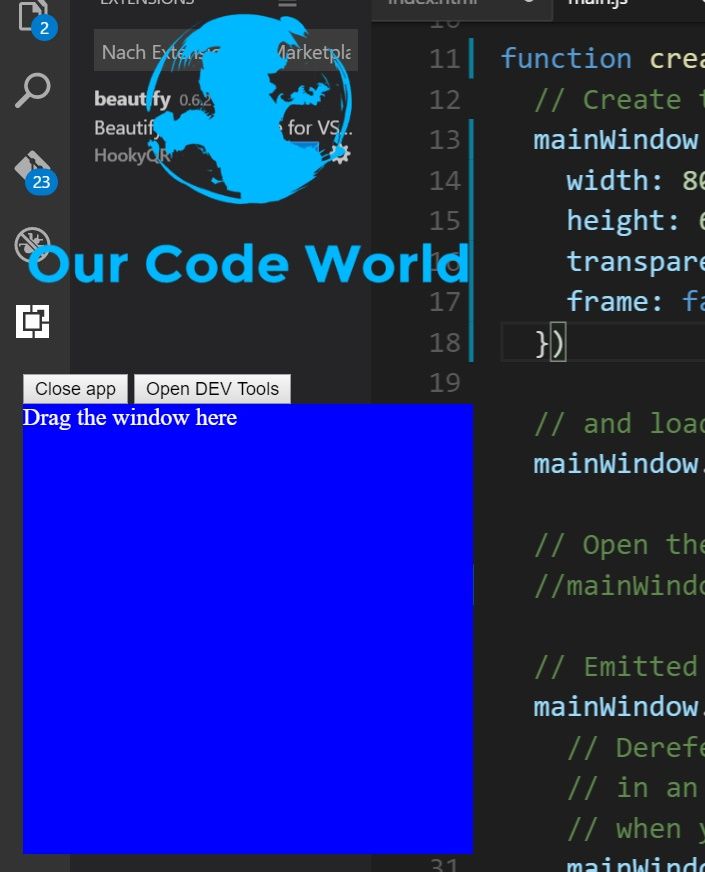
玩得开心 !
评论前必须登录!
注册