本文概述
如果你尝试在没有深入了解库的情况下自己进行操作, 则在TCPDF生成的PDF上配置”水印”可能会很棘手。幸运的是, 借助TCPDF的原始示例, 可以在可以模拟水印的PDF的任何页面上渲染背景图像。
1.覆盖TCPDF的原始Header方法
你需要做的第一件事是覆盖默认函数TCPDF Header。这种方法效果很好, 但是你将牺牲pdf的原始标头数据, 这意味着原始方法setHeaderData将不再起作用:
// This won't work anymore if you decide to add a watermark
$pdf->SetHeaderData(PDF_HEADER_LOGO, PDF_HEADER_LOGO_WIDTH, PDF_HEADER_TITLE.' 006', PDF_HEADER_STRING);
因此, 仅当你的”水印”作为整个PDF的背景播放时才建议使用此方法, 例如, 包括页眉, 水印和页脚。如果仍要继续, 请首先覆盖TCPDF类的Header方法。
这可以通过简单地在任意位置创建新类并使用其名称(只要以后可以在需要时使用它)来扩展TCPDF类来完成。这样, 你将需要存储PDF的某些原始值, 以便在使用Image方法绘制图像后将其恢复。从理论上讲, 你可以简单地复制以下示例并将路径更改为将用作水印的图像, 但是你可能想要更改将要渲染图像的边距和坐标:
class MyCustomPDFWithWatermark extends TCPDF {
public function Header() {
// Get the current page break margin
$bMargin = $this->getBreakMargin();
// Get current auto-page-break mode
$auto_page_break = $this->AutoPageBreak;
// Disable auto-page-break
$this->SetAutoPageBreak(false, 0);
// Define the path to the image that you want to use as watermark.
$img_file = './your-watermark.jpg';
// Render the image
$this->Image($img_file, 0, 0, 223, 280, '', '', '', false, 300, '', false, false, 0);
// Restore the auto-page-break status
$this->SetAutoPageBreak($auto_page_break, $bMargin);
// Set the starting point for the page content
$this->setPageMark();
}
}
2.使用新类代替TCPDF
接下来, 除了将要使用的TCPDF实例之外, 你仅需要以与以往相同的方式来生成PDF。使用替代的类而不是原始类来创建PDF实例:
// If you want to use it without Watermark
// $pdf = new TCPDF(PDF_PAGE_ORIENTATION, PDF_UNIT, PDF_PAGE_FORMAT, true, 'UTF-8', false);
// To use with watermark, use the overrided class that expects the same parameters that TCPDF
// because the class simply extends the original
$pdf = new MyCustomPDFWithWatermark(PDF_PAGE_ORIENTATION, PDF_UNIT, PDF_PAGE_FORMAT, true, 'UTF-8', false);
// Rest of your code here
// $pdf->AddPage();
就是这样, 现在应该将MyCustomPDFWithWatermark方法中定义的图像呈现在你的内容后面, 而不会出现任何视觉障碍。
完整的例子
在此示例中, 我们的PDF水印将如下所示(请注意, 这不是图像的原始尺寸, 原始尺寸与印象的尺寸1763×2162相同):
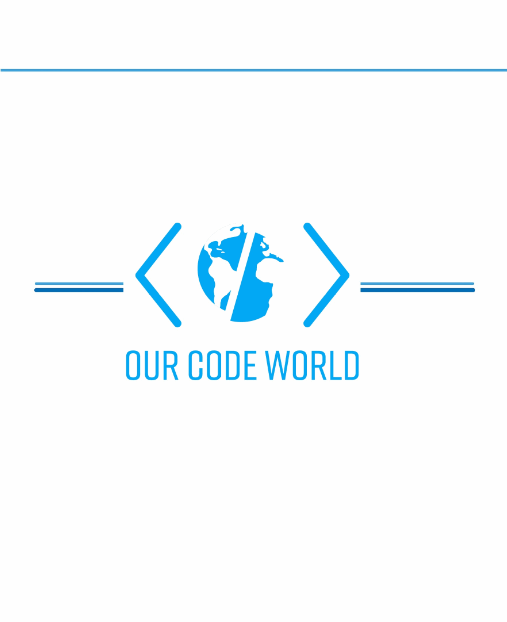
我们生成PDF的代码如下:
<?php
// Create a new PDF but using our own class that extends TCPDF
$pdf = new MyCustomPDFWithWatermark(PDF_PAGE_ORIENTATION, PDF_UNIT, PDF_PAGE_FORMAT, true, 'UTF-8', false);
// set document information
$pdf->SetAuthor('Our Code World');
// set default monospaced font
$pdf->SetDefaultMonospacedFont(PDF_FONT_MONOSPACED);
// set default header data
$pdf->SetHeaderData(PDF_HEADER_LOGO, PDF_HEADER_LOGO_WIDTH, PDF_HEADER_TITLE.' 006', PDF_HEADER_STRING);
// set margins
$pdf->SetMargins('10', '40', '10');
$pdf->SetAutoPageBreak(TRUE, PDF_MARGIN_BOTTOM);
$pdf->SetFont('dejavusans', '', 10);
// Add a page
$pdf->AddPage();
// Create some HTML content
$html = '<h1>HTML Example</h1>
Some special characters: < € € € & è è © > \\slash \\\\double-slash \\\\\\triple-slash
<h2>List</h2>
List example:
<ol>
<li><b>bold text</b></li>
<li><i>italic text</i></li>
<li><u>underlined text</u></li>
<li><b>b<i>bi<u>biu</u>bi</i>b</b></li>
<li><a href="http://www.tecnick.com" dir="ltr">link to http://www.tecnick.com</a></li>
<li>Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo.<br />Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt.</li>
<li>SUBLIST
<ol>
<li>row one
<ul>
<li>sublist</li>
</ul>
</li>
<li>row two</li>
</ol>
</li>
<li><b>T</b>E<i>S</i><u>T</u> <del>line through</del></li>
<li><font size="+3">font + 3</font></li>
<li><small>small text</small> normal <small>small text</small> normal <sub>subscript</sub> normal <sup>superscript</sup> normal</li>
</ol>
<dl>
<dt>Coffee</dt>
<dd>Black hot drink</dd>
<dt>Milk</dt>
<dd>White cold drink</dd>
</dl>
<div style="text-align:center">IMAGES
</div>';
// output the HTML content
$pdf->writeHTML($html, true, false, true, false, '');
// output some RTL HTML content
$html = '<div style="text-align:center">The words “<span dir="rtl">מזל [mazel] טוב [tov]</span>” mean “Congratulations!”</div>';
$pdf->writeHTML($html, true, false, true, false, '');
// test some inline CSS
$html = '<p>This is just an example of html code to demonstrate some supported CSS inline styles.
<span style="font-weight: bold;">bold text</span>
<span style="text-decoration: line-through;">line-trough</span>
<span style="text-decoration: underline line-through;">underline and line-trough</span>
<span style="color: rgb(0, 128, 64);">color</span>
<span style="background-color: rgb(255, 0, 0); color: rgb(255, 255, 255);">background color</span>
<span style="font-weight: bold;">bold</span>
<span style="font-size: xx-small;">xx-small</span>
<span style="font-size: x-small;">x-small</span>
<span style="font-size: small;">small</span>
<span style="font-size: medium;">medium</span>
<span style="font-size: large;">large</span>
<span style="font-size: x-large;">x-large</span>
<span style="font-size: xx-large;">xx-large</span>
</p>';
$pdf->writeHTML($html, true, false, true, false, '');
// Close and output PDF document
$pdf->Output('example_pdf_ocw.pdf', 'I');
运行代码将生成以下PDF:
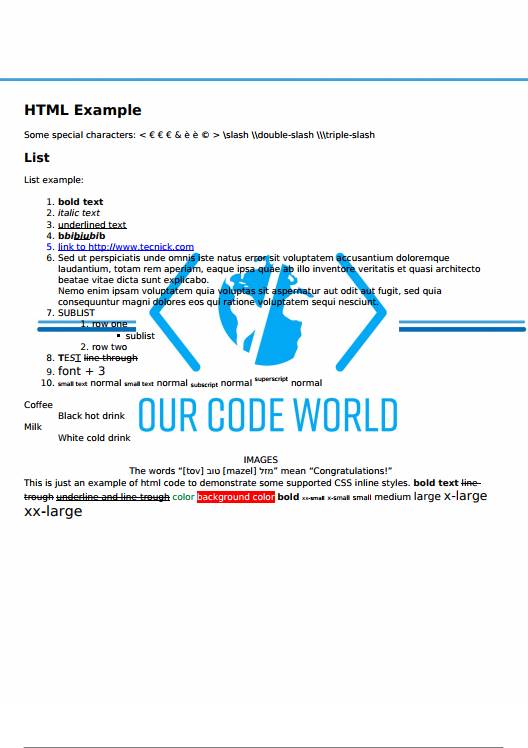
很棒而且很容易吧?编码愉快!
评论前必须登录!
注册