本文概述
当然, 与Winforms一起工作的所有C#开发人员都知道片段, 只要你知道代码, 这些片段就可以模拟任何按键的按键操作:
// Import the user32.dll
[DllImport("user32.dll", SetLastError = true)]
static extern void keybd_event(byte bVk, byte bScan, int dwFlags, int dwExtraInfo);
// Declare some keyboard keys as constants with its respective code
// See Virtual Code Keys: https://msdn.microsoft.com/en-us/library/dd375731(v=vs.85).aspx
public const int KEYEVENTF_EXTENDEDKEY = 0x0001; //Key down flag
public const int KEYEVENTF_KEYUP = 0x0002; //Key up flag
public const int VK_RCONTROL = 0xA3; //Right Control key code
// Simulate a key press event
keybd_event(VK_RCONTROL, 0, KEYEVENTF_EXTENDEDKEY, 0);
keybd_event(VK_RCONTROL, 0, KEYEVENTF_KEYUP, 0);
但是, 这可能会让人头痛。这就是为什么在本文中, 我们将向你展示使用InputSimulator模拟按键事件的正确方法。 Windows输入模拟器提供了一个简单的.NET(C#)接口, 以使用Win32 SendInput方法模拟键盘或鼠标输入。所有Interop都已为你完成, 并且有一个简单的编程模型可以发送多个击键。
使用InputSimulator的优点
Windows窗体提供了SendKeys方法, 该方法可以模拟文本输入, 但不能模拟实际的击键。 Windows输入模拟器可用于WPF, Windows窗体和控制台应用程序中, 以合成或模拟任何键盘输入, 包括控件, Alt, Shift, Tab, Enter, 空格, Backspace, Windows键, Caps Lock, Num Lock, Scroll Lock, Volume上/下和静音, Web, 邮件, 搜索, 收藏夹, 功能键, 后退和前进导航键, 可编程键以及虚拟键表中定义的任何其他键。它提供了一个简单的API, 可以模拟文本输入, 按下键, 按下键, 按下按键以及复杂的修改后的按键和弦。
想开始使用该库吗?然后继续阅读!
1.安装InputSimulator
首先需要轻松模拟按键和击键的方法是通过nuGet将库安装在项目中。打开你的Winforms C#项目, 然后在解决方案资源管理器中打开NuGet程序包管理器:
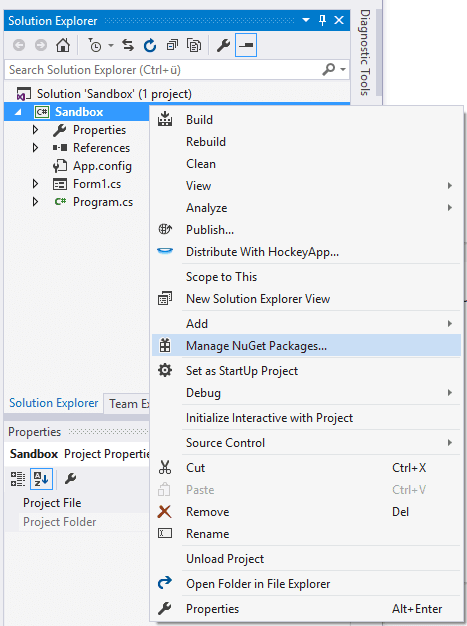
转到浏览选项卡并搜索InputSimulator:
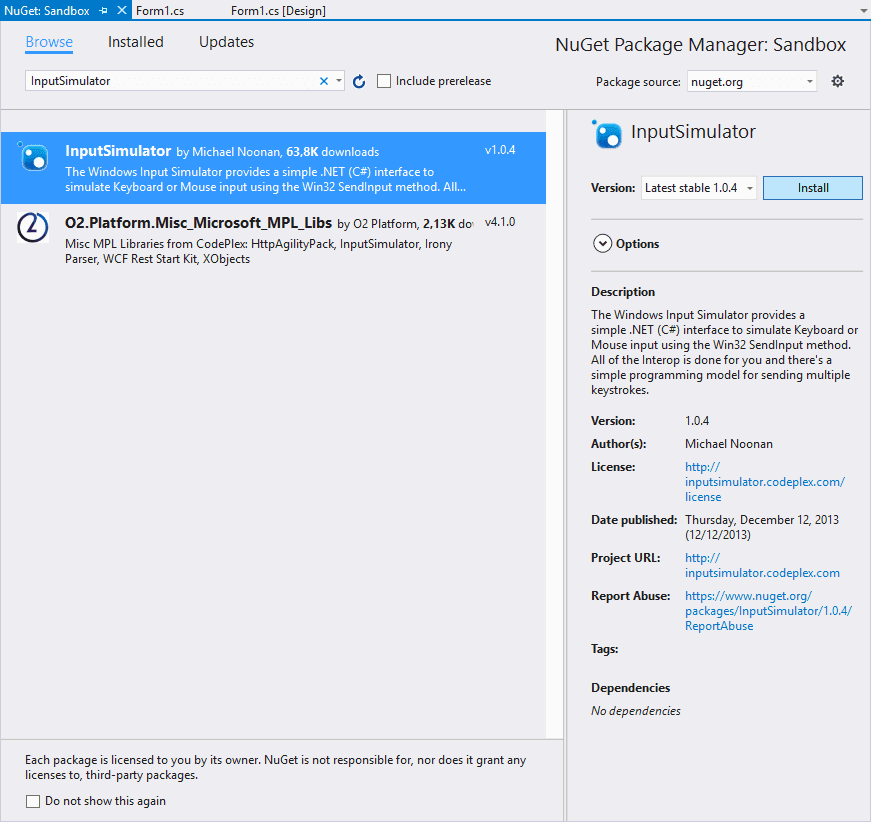
从列表中选择Michael Noonan的软件包并安装。软件包安装完成后, 你将可以在课堂上使用它。有关此库和示例的更多信息, 请访问Github上的官方存储库。
2.使用InputSimulator
在使用Input Simulator之前, 你需要在类的顶部包括所需的类型:
using WindowsInput.Native;
using WindowsInput;
虚拟代码清单
如果要模拟任何键的keypress事件, 则需要可以在此列表中获得的键码。但是, 你可以简单地使用WindowsInput.Native.VirtualKeyCode的enum属性, 该属性具有每个键的属性。
模拟按键
要模拟单个按键事件, 请使用Keyboard.KeyPress方法, 该方法需要你要模拟的按键的虚拟按键代码:
InputSimulator sim = new InputSimulator();
// Press 0 key
sim.Keyboard.KeyPress(VirtualKeyCode.VK_0);
// Press 1
sim.Keyboard.KeyPress(VirtualKeyCode.VK_1);
// Press b
sim.Keyboard.KeyPress(VirtualKeyCode.VK_B);
// Press v
sim.Keyboard.KeyPress(VirtualKeyCode.VK_V);
// Press enter
sim.Keyboard.KeyPress(VirtualKeyCode.RETURN);
// Press Left CTRL button
sim.Keyboard.KeyPress(VirtualKeyCode.LCONTROL);
如前所述, 此逻辑不仅限于单个按键事件, 对于KeyDown和KeyUp也是如此。
模拟按键
如果要模拟按键或键盘快捷方式, 可以使用键盘的ModifiedKeyStroke功能:
InputSimulator sim = new InputSimulator();
// CTRL + C (effectively a copy command in many situations)
sim.Keyboard.ModifiedKeyStroke(VirtualKeyCode.CONTROL, VirtualKeyCode.VK_C);
// You can simulate chords with multiple modifiers
// For example CTRL + K + C whic is simulated as
// CTRL-down, K, C, CTRL-up
sim.Keyboard.ModifiedKeyStroke(VirtualKeyCode.CONTROL, new[] {
VirtualKeyCode.VK_K, VirtualKeyCode.VK_C
});
// You can simulate complex chords with multiple modifiers and key presses
// For example CTRL-ALT-SHIFT-ESC-K which is simulated as
// CTRL + down, ALT + down, SHIFT + down, press ESC, press K, SHIFT-up, ALT-up, CTRL-up
sim.Keyboard.ModifiedKeyStroke(
new[] { VirtualKeyCode.CONTROL, VirtualKeyCode.MENU, VirtualKeyCode.SHIFT }, new[] { VirtualKeyCode.ESCAPE, VirtualKeyCode.VK_K }
);
输入整个单词
键盘的TextEntry方法通过键盘模拟不间断的文本输入:
InputSimulator Simulator = new InputSimulator();
Simulator.Keyboard.TextEntry("Hello World !");
模拟器API是可链接的, 因此你可以使用Sleep方法在启动之前或键入内容后等待几毫秒:
InputSimulator Simulator = new InputSimulator();
// Wait a second to start typing
Simulator.Keyboard.Sleep(1000)
// Type Hello World
.TextEntry("Hello World !")
// Wait another second
.Sleep(1000)
// Type More text
.TextEntry("Type another thing")
;
模拟按字符键入文本
如果你很懒惰, 并且想要创建使你的生活更轻松的代码片段, 则可能需要创建某种类型的roboscript, 为你编写一些文本。显然, 文本必须已经存在, 因此可以在不能键入错误的视频等项目中使用:
/// <summary>
/// Simulate typing of any text as you do when you write.
/// </summary>
/// <param name="Text">Text that will be written automatically by simulation.</param>
/// <param name="typingDelay">Time in ms to wait after 1 character is written.</param>
/// <param name="startDelay"></param>
private void simulateTypingText(string Text, int typingDelay = 100, int startDelay = 0)
{
InputSimulator sim = new InputSimulator();
// Wait the start delay time
sim.Keyboard.Sleep(startDelay);
// Split the text in lines in case it has
string[] lines = Text.Split(new string[] { "\r\n", "\n" }, StringSplitOptions.None);
// Some flags to calculate the percentage
int maximum = lines.Length;
int current = 1;
foreach (string line in lines)
{
// Split line into characters
char[] words = line.ToCharArray();
// Simulate typing of the char i.e: a, e , i , o , u etc
// Apply immediately the typing delay
foreach (char word in words)
{
sim.Keyboard.TextEntry(word).Sleep(typingDelay);
}
float percentage = ((float)current / (float)maximum) * 100;
current++;
// Add a new line by pressing ENTER
// Return to start of the line in your editor with HOME
sim.Keyboard.KeyPress(VirtualKeyCode.RETURN);
sim.Keyboard.KeyPress(VirtualKeyCode.HOME);
// Show the percentage in the console
Console.WriteLine("Percent : {0}", percentage.ToString());
}
}
该方法期望将要像人类键入的文本作为第一个参数。默认的键入延迟为100毫秒, 通常是每次按键后人类的键入延迟。最后一个参数是可选的, 仅在开始输入时提供时间延迟:
// Simulate typing text of a textbox multiline
simulateTypingText(textBox1.Text);
// Simulate typing slowly by waiting half second after typing every character
simulateTypingText(textBox1.Text, 500);
// Simulate typing slowly by waiting half second after typing every character
// and wait 5 seconds before starting
simulateTypingText(textBox1.Text, 500, 5000);
编码愉快!
评论前必须登录!
注册