本文概述
以与其他编程语言相同的方式使用PDF, 而使用C#则并非如此。可惜的是, 大多数图书馆都是商业性的, 你需要支付许可费用。 Spire PDF等其他库提供了该库的免费版本, 但是每个PDF仅支持多达10页, 然后你会看到一条水印消息, 仅用于测试目的。
如果你愿意在不使用付费API的情况下从Winforms应用程序中打印PDF, 我们将向你展示2种解决方法, 它们将帮助你轻松地打印PDF文件。
A.使用Adobe Acrobat
第一种方法要求你的用户已安装Adobe Acrobat。通常, 每台计算机都有一个读取PDF文件的程序, 即Acrobat Reader, 因此请确保检查用户是否已安装此PDF阅读器。
建议使用此方法, 因为它允许用户通过本机打印对话框选择要打印的页面, 要使用的打印机以及其他设置:
using System.Diagnostics;
using System.Threading;
public void printPDFWithAcrobat()
{
string Filepath = @"C:\Users\sdkca\Desktop\path-to-your-pdf\pdf-sample.pdf";
using (PrintDialog Dialog = new PrintDialog())
{
Dialog.ShowDialog();
ProcessStartInfo printProcessInfo = new ProcessStartInfo()
{
Verb = "print", CreateNoWindow = true, FileName = Filepath, WindowStyle = ProcessWindowStyle.Hidden
};
Process printProcess = new Process();
printProcess.StartInfo = printProcessInfo;
printProcess.Start();
printProcess.WaitForInputIdle();
Thread.Sleep(3000);
if (false == printProcess.CloseMainWindow())
{
printProcess.Kill();
}
}
}
此代码在隐藏的命令行中运行print命令, 并显示系统的”打印”对话框:

在某些Windows版本中, Acrobat Reader可能会启动一秒钟, 但在你单击”确定”后它将自动关闭, 并且文件将被打印。
B.使用RawPrint软件包
如果你不想使用Acrobat Reader, 则可能要使用将文件直接发送到打印机的自定义程序包。我们正在谈论RawPrint软件包。 RawPrint是一个绕过打印机驱动程序直接将文件发送到Windows打印机的库, 它可以将PostScript, PCL或其他打印文件类型直接发送到打印机。
要在你的项目上安装此库, 可以使用Visual Studio的NuGet包管理器。转到Visual Studio中项目的解决方案资源管理器, 然后右键单击你的项目, 从列表中选择”管理NuGet包”选项:
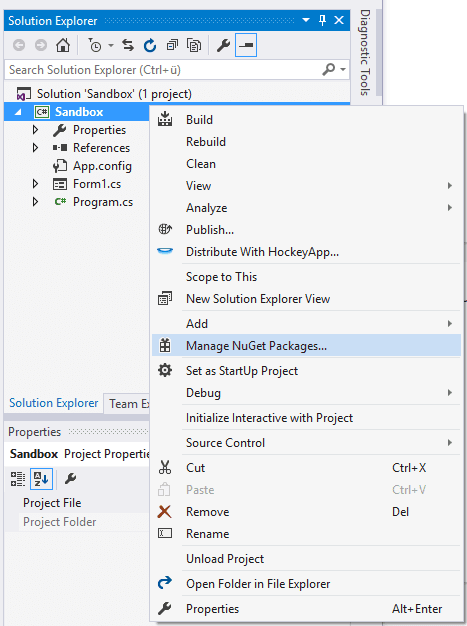
管理器显示后, 搜索RawPrint库, 选择(可能)选择Tony Edgecombe的第一个选项, 然后单击安装:

安装后, 你将可以在项目中使用RawPrint类。有关此库的更多信息, 请访问Github上的官方存储库。
1.列出打印机名称
建议在某个组合框中列出所有可用的打印机, 因此用户只需要选择所需的打印机, 然后进行打印即可。你可以使用PrinterSettings类型中的InstalledPrinters属性列出所有可用的打印机:
foreach (string printerName in System.Drawing.Printing.PrinterSettings.InstalledPrinters)
{
Console.WriteLine(printerName);
}
/** Outputs something like:
Send To OneNote 2016
Microsoft XPS Document Writer
Microsoft Print to PDF
Fax
Brother HL-3172CDW series Printer
*/
要使用RawPrint方法, 你需要指定要使用的打印机。指定使用哪个名称的唯一方法是名称, 因此在发送文件进行打印之前, 请确保具有打印机的名称。在我们的例子中, 我们有一个真正的Brother打印机, 因此在本示例中将使用它。
2.打印PDF
要从文件中打印PDF, 只需要使用RawPrint实例中的PrintRawFile方法。此方法将第一个参数用作要用于打印文件的打印机的名称, 第二个参数用作要打印的PDF文件的绝对路径(包括文件名)作为第二个参数, 最后一个参数应作为第一个参数。同一文件。最后一个参数仅用于在队列中将其显示为名称:
// Include the namespace of the library
using RawPrint;
/// <summary>
/// Prints a PDF using its RAW data directly to the printer. It requires the nuGET package RawPrint
/// </summary>
public void printPDF()
{
// Absolute path to your PDF to print (with filename)
string Filepath = @"C:\Users\sdkca\Desktop\path-to-file\pdf-sample.pdf";
// The name of the PDF that will be printed (just to be shown in the print queue)
string Filename = "pdf-sample.pdf";
// The name of the printer that you want to use
// Note: Check step 1 from the B alternative to see how to list
// the names of all the available printers with C#
string PrinterName = "Brother HL-3172CDW series Printer";
// Create an instance of the Printer
IPrinter printer = new Printer();
// Print the file
printer.PrintRawFile(PrinterName, Filepath, Filename);
}
如果执行前面的代码并且文件存在, 则打印机现在应该执行其作业。
编码愉快!
评论前必须登录!
注册