本文概述
对于Web开发人员而言, 从HTML格式创建PDF容易得多, 基本上是因为它提供了更好的管理PDF的选项。对于此任务, Web开发人员使用wkhtmltopdf之类的库。 wkhtmltopdf是使用QT Webkit呈现引擎将HTML呈现为PDF的命令行工具。它们完全”无头运行”, 不需要显示或显示服务。
在本文中, 你将学习如何在Windows Forms应用程序中轻松地将wkhtmltopdf与C#一起使用。
要求
- 你将需要带有NuGet软件包管理器(> = 2010)的Visual Studio。
在我们的例子中, 我们将使用Visual Studio社区, 所以让我们开始吧!
1.安装Pechkin
为了使用Windows窗体中的C#从HTML文件生成PDF, 我们将使用Pechkin(用于wkhtmltopdf DLL的.NET包装器), 该库使用Webkit引擎将HTML页面转换为PDF。 Pechkin作为NuGet软件包提供, 在大多数情况下, 你应该使用SynchronizedPechkin, 因为它可以保护多线程代码免遭lib崩溃。但是对于一个线程的简单使用, 你可以直接使用SimplePechkin。
注意
Pechkin在项目(和NuGet包)中包括最新版本的wkhtmltox DLL及其依赖项, 并复制到项目构建的build文件夹中。因此, 在计算机上使用该库之前, 无需安装任何先决条件。
通过访问NuGET程序包管理器, 使用Visual Studio安装程序包。在Visual Studio右上方的解决方案资源管理器中右键单击你的项目, 然后在下拉菜单中选择”管理NuGet程序包”:
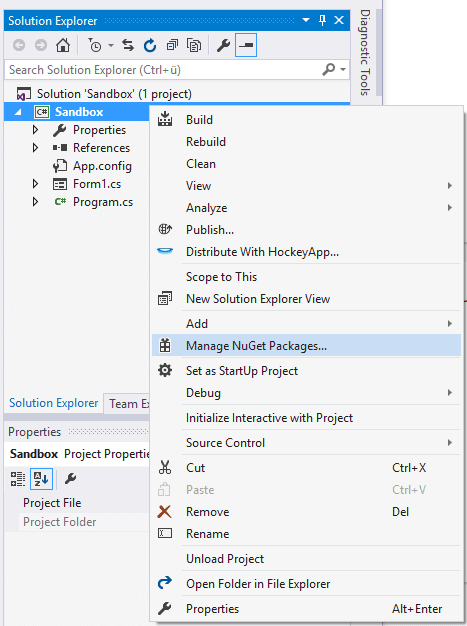
现在, 从紧急窗口中搜索Pechkin.Synchronized包, 选择它并将其安装在你的项目中:
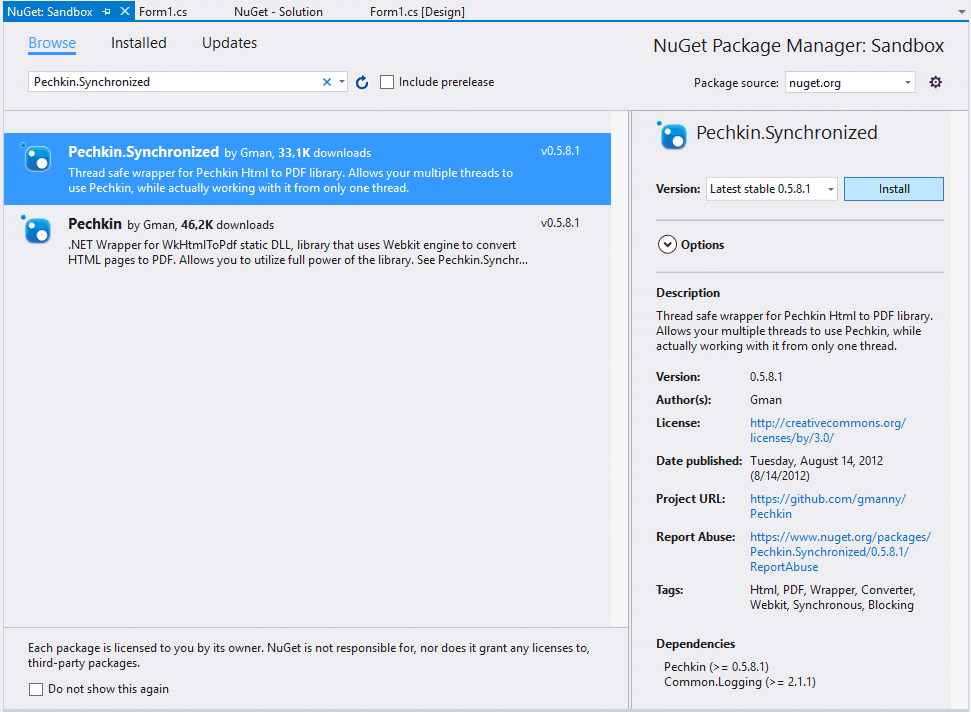
设置完成后, 你将可以在项目中使用包装器。
2.生成PDF
Convert方法使用给定的设置将HTML字符串(或配置对象)转换为PDF, 并返回存储PDF二进制数据的字节数组。
注意
对于所有示例, pdfContent变量将仅生成PDF的内容, 你将看不到任何内容, 因此请不要忘记按照步骤3将创建的内容写入文件。
A.从HTML字符串生成简单的PDF
你可以使用空的全局配置对象快速创建PDF。在你的课程中导入Pechkin类型:
using Pechkin;
然后使用以下代码段创建PDF的内容:
byte[] pdfContent = new SimplePechkin(new GlobalConfig()).Convert("<html><body><h1>Hello world!</h1></body></html>");
B.从网站生成PDF
你可以使用配置中的SetPageUri方法通过其URL呈现网站, 而不是普通的HTML字符串。在类的顶部导入Pechkin.Synchronized类型:
using Pechkin.Synchronized;
然后使用以下代码从网站创建PDF:
// create global configuration object
GlobalConfig gc = new GlobalConfig();
// set it up using fluent notation
// Remember to import the following type:
// using System.Drawing.Printing;
//
// a new instance of Margins with 1-inch margins.
gc.SetMargins(new Margins(100, 100, 100, 100))
.SetDocumentTitle("Test document")
.SetPaperSize(PaperKind.Letter)
// Set to landscape
//.SetPaperOrientation(true)
;
// Create converter
IPechkin pechkin = new SynchronizedPechkin(gc);
// Create document configuration object
ObjectConfig configuration = new ObjectConfig();
// and set it up using fluent notation too
configuration.SetCreateExternalLinks(false)
.SetFallbackEncoding(Encoding.ASCII)
.SetLoadImages(true)
.SetPageUri("http://ourcodeworld.com");
// Generate the PDF with the given configuration
// The Convert method will return a Byte Array with the content of the PDF
// You will need to use another method to save the PDF (mentioned on step #3)
byte[] pdfContent = pechkin.Convert(configuration);
C.从本地HTML文件生成PDF
要从本地HTML文件创建PDF, 可以通过使用SetPageUri方法(使用网站的方式)为文件的本地文件路径提供文件, 但同时允许使用本地资源使用SetAllowLocalContent方法(请注意, 你需要将前缀file:///附加到html文件路径中)。在类的顶部导入Pechkin.Synchronized类型:
using Pechkin.Synchronized;
并使用以下代码从本地HTML文件创建PDF:
// create global configuration object
GlobalConfig gc = new GlobalConfig();
// set it up using fluent notation
// Remember to import the following type:
// using System.Drawing.Printing;
//
// a new instance of Margins with 1-inch margins.
gc.SetMargins(new Margins(100, 100, 100, 100))
.SetDocumentTitle("Test document")
.SetPaperSize(PaperKind.Letter);
// Create converter
IPechkin pechkin = new SynchronizedPechkin(gc);
// Create document configuration object
ObjectConfig configuration = new ObjectConfig();
string HTML_FILEPATH = "C:/Users/sdkca/Desktop/example.html";
// and set it up using fluent notation too
configuration
.SetAllowLocalContent(true)
.SetPageUri(@"file:///" + HTML_FILEPATH);
// Generate the PDF with the given configuration
// The Convert method will return a Byte Array with the content of the PDF
// You will need to use another method to save the PDF (mentioned on step #3)
byte[] pdfContent = pechkin.Convert(configuration);
现在你知道了如何生成PDF的内容, 你只需要学习如何保存它即可。
3.保存PDF
如前所述, Pechkin生成PDF并将数据作为字节数组返回, 这意味着你将需要自己编写数据。我们将使用程序集System.IO中包含的FileStream类将PDF写入文件。使用FileStream, 你可以读取, 写入, 打开和关闭文件系统上的文件, 还可以操纵其他与文件相关的操作系统句柄, 包括管道, 标准输入和标准输出。在使用它之前, 请在类顶部使用using指令导入以下类型:
using System.IO;
然后, 使用该类创建一个以返回的ByteArray作为内容的文件。你可以使用以下方法将文件的绝对路径作为第一个参数, 并将要写入的数据作为第二个参数(pdf内容):
/// <summary>
/// Writes a byte array (format returned by SimplePechkin) into a file
/// </summary>
/// <param name="_FileName"></param>
/// <param name="_ByteArray"></param>
/// <returns></returns>
public bool ByteArrayToFile(string _FileName, byte[] _ByteArray)
{
try
{
// Open file for reading
FileStream _FileStream = new FileStream(_FileName, FileMode.Create, FileAccess.Write);
// Writes a block of bytes to this stream using data from a byte array.
_FileStream.Write(_ByteArray, 0, _ByteArray.Length);
// Close file stream
_FileStream.Close();
return true;
}
catch (Exception _Exception)
{
Console.WriteLine("Exception caught in process while trying to save : {0}", _Exception.ToString());
}
return false;
}
然后可以如以下示例所示使用它:
// Simple PDF from String
byte[] pdfBuffer = new SimplePechkin(new GlobalConfig()).Convert("<html><body><h1>Hello world!</h1></body></html>");
// Folder where the file will be created
string directory = "C:\\Users\\sdkca\\Desktop\\";
// Name of the PDF
string filename = "hello_world.pdf";
if (ByteArrayToFile(directory + filename, pdfBuffer))
{
Console.WriteLine("PDF Succesfully created");
}
else
{
Console.WriteLine("Cannot create PDF");
}
编码愉快!
评论前必须登录!
注册