本文概述
QR码是一种流行的二维条形码。它们也称为硬链接或物理世界超链接。 QR码最多可存储4, 296个任意文本的字母数字字符。该文本可以是任何内容, 例如URL, 联系信息, 电话号码, 甚至是一首诗!光学设备可以使用相应的软件读取QR码。这样的设备范围从专用的QR码阅读器到移动电话。
不管怎样, 每个人都知道QR码是什么, 但是比带有你自己的徽标的自定义QR码更酷的是什么?使用PHP, 此任务并非易事, 但并非不可能。
有两种方法可以实现你的目标, 或者使用生成QR码的库并允许你对其进行自定义(可以自定义徽标), 也可以使用php自行实现, 你可以在另一个图像上呈现图像, 这意味着我们将在已经生成的QR码图像上渲染徽标。
1.使用库
库使你的生活更轻松, 在这种情况下, 要在QR Code中实现自定义徽标, 可以使用Endroid QRCode包。该库基于Y. Swetake的QRcode Perl CGI和PHP脚本, 它可以帮助你生成包含QR码的图像。
安装此库的首选方法是通过composer进行安装, 请执行以下composer命令:
composer require endroid/qrcode
或手动编辑composer.json并执行composer安装:
{
"require": {
"endroid/qrcode": "^1.7", }
}
现在, 在安装之后, 在你的类中包含带有use语句的类:
use Endroid\QrCode\QrCode;
然后使用以下代码创建第一个带有徽标的QR代码:
返回带有徽标的QR码作为响应
// Include the QRCode generator library
use Endroid\QrCode\QrCode;
$qrCode = new QrCode();
$qrCode->setText('http://ourcodeworld.com')
->setSize(300)
->setPadding(10)
->setErrorCorrection('high')
->setForegroundColor(array('r' => 0, 'g' => 0, 'b' => 0, 'a' => 0))
->setBackgroundColor(array('r' => 255, 'g' => 255, 'b' => 255, 'a' => 0))
// Path to your logo with transparency
->setLogo("logo.png")
// Set the size of your logo, default is 48
->setLogoSize(98)
->setImageType(QrCode::IMAGE_TYPE_PNG)
;
// Send output of the QRCode directly
header('Content-Type: '.$qrCode->getContentType());
$qrCode->render();
将带有徽标的QR代码保存在服务器中
// Include the QRCode generator library
use Endroid\QrCode\QrCode;
$qrCode = new QrCode();
$qrCode->setText('http://ourcodeworld.com')
->setSize(300)
->setPadding(10)
->setErrorCorrection('high')
->setForegroundColor(array('r' => 0, 'g' => 0, 'b' => 0, 'a' => 0))
->setBackgroundColor(array('r' => 255, 'g' => 255, 'b' => 255, 'a' => 0))
// Path to your logo with transparency
->setLogo("logo.png")
// Set the size of your logo, default is 48
->setLogoSize(98)
->setImageType(QrCode::IMAGE_TYPE_PNG)
;
$qrCode->save('qrcode.png');
任何上述方法的执行均应生成以下QRCode:
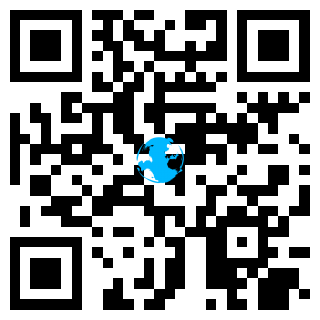
不要忘记为徽标设置透明的有效路径, 如你所见, Endroid QRCode提供了许多有用的方法, 因为自定义标签可能对你有用, 请访问存储库以获取更多信息。
2.手动执行
此功能是可靠的, 因为即使中间有少量图像, QR码仍然可以读取。
快速示例
在这种情况下, 我们将使用一个简单的代码段, 该代码段将从Google的Chart API中检索QR码, 并返回生成的QR码。
由于其简单性, (以我为例)是最简单的解决方案。
<?php
// Text content of the QRCode
$data = 'http://ourcodeworld.com';
// QRCode size
$size = '500x500';
// Path to image (web or local)
$logo = 'http://ourcodeworld.com/resources/img/ocw-empty.png';
// Get QR Code image from Google Chart API
// http://code.google.com/apis/chart/infographics/docs/qr_codes.html
$QR = imagecreatefrompng('https://chart.googleapis.com/chart?cht=qr&chld=H|1&chs='.$size.'&chl='.urlencode($data));
// START TO DRAW THE IMAGE ON THE QR CODE
$logo = imagecreatefromstring(file_get_contents($logo));
$QR_width = imagesx($QR);
$QR_height = imagesy($QR);
$logo_width = imagesx($logo);
$logo_height = imagesy($logo);
// Scale logo to fit in the QR Code
$logo_qr_width = $QR_width/3;
$scale = $logo_width/$logo_qr_width;
$logo_qr_height = $logo_height/$scale;
imagecopyresampled($QR, $logo, $QR_width/3, $QR_height/3, 0, 0, $logo_qr_width, $logo_qr_height, $logo_width, $logo_height);
// END OF DRAW
/**
* As this example is a plain PHP example, return
* an image response.
*
* Note: you can save the image if you want.
*/
header('Content-type: image/png');
imagepng($QR);
imagedestroy($QR);
// If you decide to save the image somewhere remove the header and use instead :
// $savePath = "/path/to-my-server-images/myqrcodewithlogo.png";
// imagepng($QR, $savePath);
?>
注意:上一个代码片段将生成与本文图像相同的QR代码, 你可以在PHPFiddle中对其进行测试, 也可以在你的环境中对其进行本地测试。如果需要, 你可以优化方法以使用更复杂的方法来创建对Google网站的请求, 而不是使用file_get_contents。
基本故障排除
徽标是完全透明的, 并覆盖QR码
有时在存在透明度的情况下会发生此问题, 但它会删除QR码所在的区域, 例如:
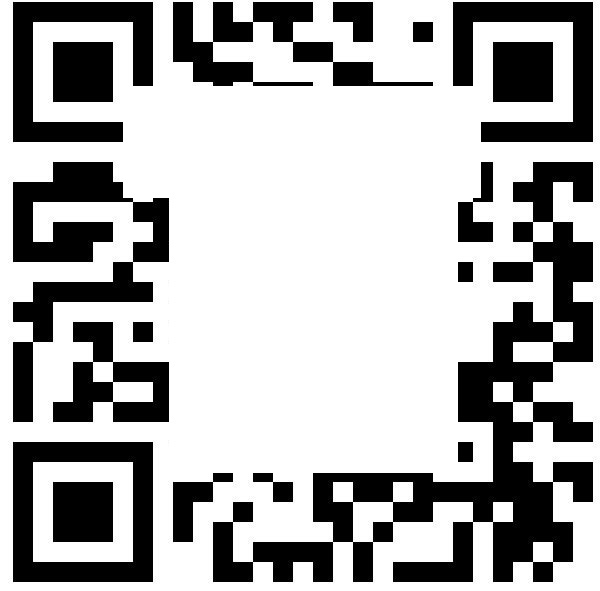
要解决它, 我们需要使用以下几行
// START TO DRAW THE IMAGE ON THE QR CODE
$logo = imagecreatefromstring(file_get_contents($logopath));
/**
* Fix for the transparent background
*/
imagecolortransparent($logo , imagecolorallocatealpha($logo , 0, 0, 0, 127));
imagealphablending($logo , false);
imagesavealpha($logo , true);
这些行将防止透明性覆盖QR码, 但是仍会根据需要使用透明性。
最后, 我们的固定代码段如下所示:
<?php
require_once("phpqrcode/qrlib.php");
// Path where the images will be saved
$filepath = 'myimage.png';
// Image (logo) to be drawn
$logopath = 'http://ourcodeworld.com/resources/img/ocw-empty.png';
// qr code content
$codeContents = 'http://ourcodeworld.com';
// Create the file in the providen path
// Customize how you want
QRcode::png($codeContents, $filepath , QR_ECLEVEL_H, 20);
// Start DRAWING LOGO IN QRCODE
$QR = imagecreatefrompng($filepath);
// START TO DRAW THE IMAGE ON THE QR CODE
$logo = imagecreatefromstring(file_get_contents($logopath));
/**
* Fix for the transparent background
*/
imagecolortransparent($logo , imagecolorallocatealpha($logo , 0, 0, 0, 127));
imagealphablending($logo , false);
imagesavealpha($logo , true);
$QR_width = imagesx($QR);
$QR_height = imagesy($QR);
$logo_width = imagesx($logo);
$logo_height = imagesy($logo);
// Scale logo to fit in the QR Code
$logo_qr_width = $QR_width/3;
$scale = $logo_width/$logo_qr_width;
$logo_qr_height = $logo_height/$scale;
imagecopyresampled($QR, $logo, $QR_width/3, $QR_height/3, 0, 0, $logo_qr_width, $logo_qr_height, $logo_width, $logo_height);
// Save QR code again, but with logo on it
imagepng($QR, $filepath);
// End DRAWING LOGO IN QR CODE
// Ouput image in the browser
echo '<img src="'.$filepath.'" />';
尽管有一个开放源码的librarie可以为你解决问题, 但是你仍然可以使用一些简单的PHP代码来实现它。
推荐建议
如你在第二种方法中看到的, 我们没有使用任何库。我们只是从Google Charts API下载QR图像响应, 并在其上绘制徽标。但是, 你可能不希望依赖服务器google, 因为此API已根据此处的主页弃用, 因此他们建议你改用主动维护的Google Charts API(不支持QR码)。
我们建议你使用开放源代码QR代码生成器库作为TCPDF(也生成PDF)或最常见和最著名的PHP QR代码。
PHP QR Code是开源(LGPL)库, 用于生成二维码QR Code。基于libqrencode C库, 提供用于创建QR Code条码图像(由于GD2而产生PNG, JPEG)的API。完全由PHP实施, 没有任何外部依赖项(如果需要, GD2除外)。
库的某些功能包括:
- 支持QR码版本(大小)1-40。
- 数字, 字母数字, 8位和汉字编码。
- 完全用PHP实现, 除GD2外没有任何外部依赖项。
- 导出为PNG, JPEG图像, 也导出为位表。
- TCPDF 2-D条码API集成。
- 易于配置。
- 数据缓存可加快计算速度。
- 提供的合并工具有助于将库作为一个大的无依赖文件进行部署, 易于”包含且不担心”。
- 调试数据转储, 错误记录, 时间基准测试。
- API文档。
- 详细的例子。
- 100%开放源代码, LGPL许可。
以下代码段显示了使用PHP QR Code的有效解决方案:
<?php
require_once("phpqrcode/qrlib.php");
// Path where the images will be saved
$filepath = 'examplefolder/myimage.png';
// Image (logo) to be drawn
$logopath = 'http://ourcodeworld.com/resources/img/ocw-empty.png';
// qr code content
$codeContents = 'http://ourcodeworld.com';
// Create the file in the providen path
// Customize how you want
QRcode::png($codeContents, $filepath , QR_ECLEVEL_H, 20);
// Start DRAWING LOGO IN QRCODE
$QR = imagecreatefrompng($filepath);
// START TO DRAW THE IMAGE ON THE QR CODE
$logo = imagecreatefromstring(file_get_contents($logopath));
$QR_width = imagesx($QR);
$QR_height = imagesy($QR);
$logo_width = imagesx($logo);
$logo_height = imagesy($logo);
// Scale logo to fit in the QR Code
$logo_qr_width = $QR_width/3;
$scale = $logo_width/$logo_qr_width;
$logo_qr_height = $logo_height/$scale;
imagecopyresampled($QR, $logo, $QR_width/3, $QR_height/3, 0, 0, $logo_qr_width, $logo_qr_height, $logo_width, $logo_height);
// Save QR code again, but with logo on it
imagepng($QR, $filepath);
// End DRAWING LOGO IN QR CODE
// Ouput image in the browser
echo '<img src="'.$filepath.'" />';
?>
注意:包括完整版本的qrlib.php(还必须提供软件包形式的所有库文件以及缓存目录)或合并版本的phpqrcode.php(仅一个文件, 但由于配置了禁用的缓存和更快的屏蔽功能, 代码速度较慢且准确性较低) )。
玩得开心 !
评论前必须登录!
注册